Bit operations for creative coding
I'm investigating the idea of converting a byte value to a tile. The idea is to use the properties of the byte to determine the properties of the tile. This could possibly be used with a grayscale image to create a mosaic of tiles.
Before I figure out an artwork to create with this concept, I want to experiment with the idea. I'll start by creating a simple grid of tiles, each tile representing a byte value.
Leveraging a bitmask to extract the properties of the byte, I could then use these properties to draw the tile. Here's a set or arbitrary rules I'll use to determine the properties of the tile based on the binary representation of the byte:
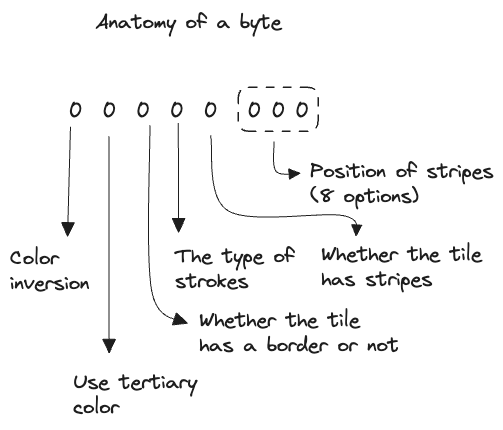
Extracting properties
Now that we have a set of rules to determine the properties of the tile, we need to extract these properties from the byte value. We can do this by using a bitmask to extract the bits that represent each property.
Example of extracting the properties from a byte value:
const variations = type & 0b111
const striped = (type & 0b1000) >> 3
const stripeType = (type & 0b10000) >> 4
const border = (type & 0b100000) >> 5
const useTertiary = (type & 0b1000000) >> 6
const inverted = (type & 0b10000000) >> 7
Some more advanced usages could include using the properties to determine the color of the tile, the shape of the tile, or the texture of the tile, etc
Rendering tiles based on properties
I want to be able to visualize the properties of the byte value by rendering a tile based on these properties. I'll start by creating a simple grid of tiles, each tile representing a byte value.
Observations
As of now, it's hard to see the patterns in the tiles. I think it would be beneficial to add some more variation to the tiles to make them more visually distinct. I don't yet have a good use case for this technique, but I believe it could be useful for generating procedural textures or patterns.
In short, to be continued...